๋ฐ์ํ
package makingTv;
public class Tv {
String modelName;
String companyName;
int price;
boolean powerState;
int currentVolume;
int maxVolume;
Tv(String modelName, String companyName, int price, int maxVolume){
this.modelName = modelName;
this.companyName = companyName;
this.price = price;
/*
* if(maxVolume <= 0){
* this.maxVolume = 10;
* }else{
* this.maxVolume = maxVolume;
* }
*/
this.maxVolume = maxVolume <= 0 ? 10 : maxVolume;
this.powerState = false;
this.currentVolume = 0;
}
// - turnOn ๋ฉ์๋ : ํฐ๋น ์ ์์ ํต๋๋ค ์ถ๋ ฅ ํ, ์ ์ ์ํ๋ฅผ ๋ณ๊ฒฝํ๋ค
void turnOn() {
System.out.println("ํฐ๋น ์ ์์ ํต๋๋ค");
powerState = true;
}
// - turnOff ๋ฉ์๋ : ํฐ๋น ์ ์์ ๋๋๋ค ์ถ๋ ฅ ํ, ์ ์ ์ํ๋ฅผ ๋ณ๊ฒฝํ๋ค
void turnOff() {
System.out.println("ํฐ๋น ์ ์์ ๋๋๋ค");
powerState = false;
}
// - volumeUp ๋ฉ์๋ : ํฐ๋น ์ ์ ์ํ๊ฐ ์ผ์ ธ ์๋ค๋ฉด, ํ์ฌ ๋ณผ๋ฅจ์ 1 ์ฆ๊ฐ์ํจํ ํ์ฌ ๋ณผ๋ฅจ์ ์ถ๋ ฅํ๋ค
// (๋จ, ํ์ฌ ๋ณผ๋ฅจ์ ์ต๋ ๋ณผ๋ฅจ์ ๋์ง ๋ชปํ๋ค)
void volumeUp() {
// ํฐ๋น ์ ์ ์ํ๊ฐ ๊บผ์ ธ ์๋ค๋ฉด ๋ฉ์๋ ์ข
๋ฃ
if(!powerState) {
System.out.println("ํฐ๋น ์ ์์ด ๊บผ์ ธ ์์ต๋๋ค");
return; // return : ์คํ๋๋ฉด ๋ฉ์๋์ ์คํ์ด ์ข
๋ฃ๋๋ค
// ์๋์ ์ฝ๋๊ฐ ์คํ๋์ง ์๋๋ค
}
// ํฐ๋น์ ํ์ฌ ๋ณผ๋ฅจ์ด ์ต๋ ๋ณผ๋ฅจ์ ๋๋๋ค๋ฉด ๋ฉ์๋ ์ข
๋ฃ
if(currentVolume >= maxVolume) {
System.out.println("ํ์ฌ ์ต๋ ๋ณผ๋ฅจ์
๋๋ค");
return;
}
// if(powerState && currentVolume < maxVolume)
currentVolume++;
System.out.println("ํ์ฌ ๋ณผ๋ฅจ : " + currentVolume);
}
// - volumeDown ๋ฉ์๋ : ํฐ๋น ์ ์ ์ํ๊ฐ ๊บผ์ ธ ์๋ค๋ฉด, ํ์ฌ ๋ณผ๋ฅจ์ 1 ๊ฐ์์ํจํ ํ์ฌ ๋ณผ๋ฅจ์ ์ถ๋ ฅํ๋ค
// (๋จ, ํ์ฌ ๋ณผ๋ฅจ์ 0๋ณด๋ค ์์์ง์ง ๋ชปํ๋ค)
void volumeDown() {
if(powerState && currentVolume > 0) {
currentVolume--;
System.out.println("ํ์ฌ ๋ณผ๋ฅจ : " + currentVolume);
}
}
์ด ์ฝ๋ฉ ๋ธ๋ญ์ Tv๋ผ๋ ํด๋์ค์ด๋ค.
package makingTv;
public class TvTest {
public static void main(String[] args) {
Tv tv1 = new Tv("์ผ์ฑํฐ๋น", "์ผ์ฑ", 1000, 5);
Tv tv2 = new Tv("์์งํฐ๋น", "์์ง", 1500, -3);
// ์์ฑ์์ ๊ฒ์ฆ ๋ก์ง์ด ์ ๋๋์ง ํ์ธ
System.out.println(tv1.powerState);
System.out.println(tv2.maxVolume);
// ์ ์์ด ๊บผ์ ธ์๋๋ฐ ๋ณผ๋ฅจ์ ๋์ด๋ ๊ฒฝ์ฐ
tv1.volumeUp();
// ์ ์์ด ๊บผ์ ธ์๋๋ฐ ๋ณผ๋ฅจ์ ๋ฎ์ถ๋ ๊ฒฝ์ฐ
tv1.volumeDown();
System.out.println(tv1.currentVolume);
// ์ ์์ด ์ผ์ ธ์๋๋ฐ ํ์ฌ ๋ณผ๋ฅจ์ด 0์ผ๋ ๋ณผ๋ฅจ์ ๋ฎ์ถ๋ ๊ฒฝ์ฐ
tv1.turnOn();
tv1.volumeDown();
System.out.println(tv1.currentVolume);
// ์ ์์ด ์ผ์ ธ์๊ณ , ํ์ฌ ๋ณผ๋ฅจ์ ๋ํ ์ ์๋ ๊ฒฝ์ฐ
tv1.volumeUp();
}
}
์ด ์ฝ๋ ๋ธ๋ญ์ ์คํ์ฐฝ์ด๋ผ๊ณ ๋ณผ ์ ์๋ ๊ฒ์ด๋ค.
์ด ์ฝ๋ฉ์ ํด๋์ค์ ๋ฉ์๋์ ์ฌ์ฉ๋ฐฉ๋ฒ์ ์๊ณ ์๋์ง ํ์ธํ๊ธฐ์ ์ข์ ์์์ด๋ค.
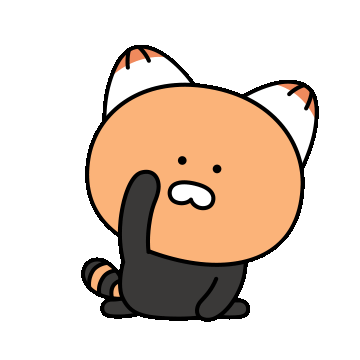
728x90
'์ธ์ด > JAVA' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
JAVA : Final(์์) ์ฌ์ฉํ๊ธฐ (0) | 2024.08.21 |
---|---|
JAVA : ์ ๊ทผ ์ ํ์์ static (0) | 2024.08.18 |
JAVA : ์์(Inheritance) (0) | 2024.08.16 |
JAVA : ๋ฉ์๋(method) (0) | 2024.08.12 |
JAVA : ์์ฑ์ (0) | 2024.08.12 |